Multiple Upload File Programmatically Using GridView
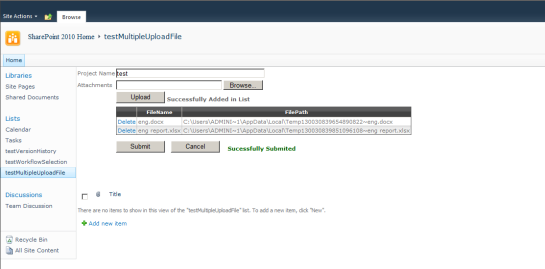
1
2
| <asp:GridView ID = "dgdUpload" runat = "server" AutoGenerateDeleteButton = "True" OnRowDeleting = "dgdUpload_RowDeleting" PagerStyle - CssClass = "pgr" AlternatingRowStyle - CssClass = "alt" CssClass = "mGrid" > < / asp:GridView> |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
| using System; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web; using System.Text; using System.Data; using System.IO; using Microsoft.SharePoint; namespace EcoMultipleFile.MultipleFileUpload { public partial class MultipleFileUploadUserControl : UserControl { private string fileName = ""; private double length; int DocNo = 0 ; DataTable dt; / / datatable use for multiple file upload DataRow dr; / / datarow use for multiple file upload DataColumn dc; / / datacolumn use for multiple file upload protected void Page_Load( object sender, EventArgs e) { } protected void dgdUpload_RowDeleting( object sender, GridViewDeleteEventArgs e) { int recordToDelete = e.RowIndex; dt = (DataTable)ViewState[ "Files" ]; int cn = dt.Rows.Count; dt.Rows.RemoveAt(recordToDelete); dt.AcceptChanges(); ViewState[ "Files" ] = dt; this.dgdUpload.DataSource = dt; this.dgdUpload.DataBind(); lblMessage.Text = " File successfully being deleted" ; } private void AddMoreColumns() { dt = new DataTable( "Files" ); / / dc = new DataColumn( "No" , Type .GetType( "System.Int16" )); / / dt.Columns.Add(dc); dc = new DataColumn( "FileName" , Type .GetType( "System.String" )); dt.Columns.Add(dc); dc = new DataColumn( "FilePath" , Type .GetType( "System.String" )); dt.Columns.Add(dc); ViewState[ "Files" ] = dt; } protected void Submit_Click( object sender, EventArgs e) { / / Get the Site collection using (SPSite oSite = new SPSite(SPContext.Current.Web.Url)) { / / Open the Root web using (SPWeb oWeb = oSite.OpenWeb()) { dt = (DataTable)ViewState[ "Files" ]; int _dtcnt = dt.Rows.Count; string strDate = ""; foreach (DataRow dr in dt.Rows) { strDate = System.DateTime.Now.Date.TimeOfDay.ToString(); / * * * Simple File Upload * * * * * * * * / / / Read the file from the stream into the byte array string strFilepath = dr[ "FilePath" ].ToString(); string fileName1 = dr[ "FileName" ].ToString(); FileStream fs = File .OpenRead(strFilepath); byte[] FileContent = new byte[fs.Length]; fs.Read(FileContent, 0 , Convert.ToInt32(fs.Length)); fs.Close(); / / Get the documents Library SPList DocLib = oWeb.Lists[ "Shared Documents" ]; / * * * If you want to add inside a folder * * * * * * * / SPFolder SubFolder = DocLib.RootFolder.SubFolders[ "TestFolder" ]; / / Add the file to the sub - folder SPFile file = SubFolder.Files.Add(SubFolder.Url + "/" + fileName1, FileContent, true); SubFolder.Update(); / / If you want to Update the Meta data column say "Project A" SPListItem item = DocLib.Items[ file .UniqueId]; item[ "Project Title" ] = TxtProjectName.Text; item.Update(); } LblSubmit.Text = "Sucessfully Submited" ; } } } protected void btnUpload_Click( object sender, EventArgs e) { fileName = System.IO.Path.GetFileName(FileUpload1.PostedFile.FileName); if (fileName ! = "") { string _fileTime = DateTime.Now.ToFileTime().ToString(); string _fileorgPath = System.IO.Path.GetFullPath(FileUpload1.PostedFile.FileName); string _newfilePath = _fileTime + "~" + fileName; length = (FileUpload1.PostedFile.InputStream.Length) / 1024 ; string tempFolder = Environment.GetEnvironmentVariable( "TEMP" ); string _filepath = tempFolder + _newfilePath; FileUpload1.PostedFile.SaveAs(_filepath); AddRow(fileName, _filepath, DocNo, true); DocNo = DocNo + 1 ; lblMessage.Text = "Successfully Added in List" ; } else { lblMessage.Text = "Select a File" ; return ; } } private void AddRow(string file , string path, int ID , Boolean bolCheckForfiles) { Boolean bolAddRow = true; dt = (DataTable)ViewState[ "Files" ]; if (dt = = null) { AddMoreColumns(); } if (bolCheckForfiles) { if (dt.Rows.Count > 0 ) { foreach (DataRow drExistingrow in dt.Rows) { if (drExistingrow[ "FileName" ].ToString() = = file ) { bolAddRow = false; } } } } if (bolAddRow) { dr = dt.NewRow(); dr[ "FileName" ] = file ; dr[ "FilePath" ] = path; dt.Rows.Add(dr); ViewState[ "Files" ] = dt; dgdUpload.DataSource = dt; dgdUpload.DataBind(); / / bind in grid } else { lblMessage.Visible = true; lblMessage.Text = "Same File Name already exists!!!" ; } } } } |
No comments:
Post a Comment